How to Debug Stateful Frontend Apps Like a Pro
Learn the art of debugging stateful frontend apps by harnessing the power of reset flags in your app's URL. Discover how this technique can streamline testing and ensure a seamless user experience.
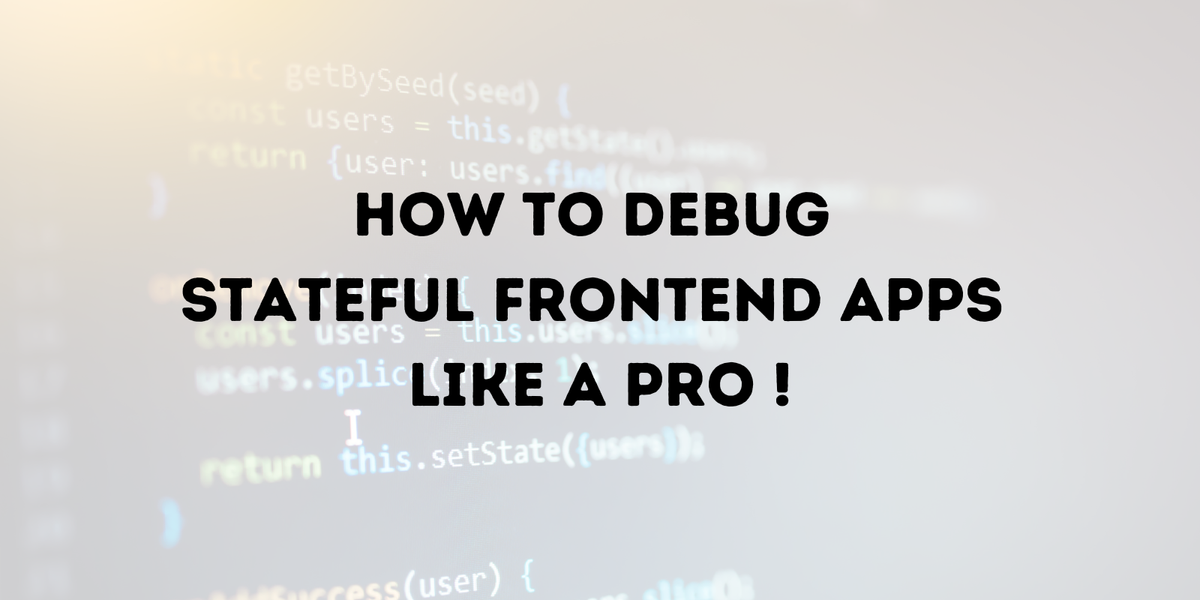
When you're working on front-end applications that rely heavily on state management, debugging can be quite a challenge. Fortunately, there's a powerful technique that can make your life easier and help you spot and fix issues before they impact your users. In this article, we'll explore how to debug stateful front-end apps effectively.
In modern web development, it's common to store application states in local storage or similar storage libraries. This practice is crucial for enhancing frontend app performance, as it reduces the need to constantly fetch data from external APIs.
However, as a front-end developer, you know that thoroughly testing and debugging stateful apps is crucial to ensure they work as expected. Here's where the concept of 'reset flags' comes into play. A reset flag is a special parameter added to your app's URL, and it can be a game-changer for debugging.
So, how does it work? Let's break it down:
- Setting the Stage: Imagine you're working on a complex frontend app with various states. You want to make sure that a new feature or change you're implementing doesn't interfere with existing functionality. This is where a reset flag becomes your best friend.
- Adding the Reset Flag: To initiate a full reset of all stored states in your app, you add a reset flag to the URL. This flag acts as a trigger, telling your app to start from scratch when it loads.
- Testing with a Clean Slate: After adding the reset flag to the URL, simply refresh the page. Your app will now load as if it were brand new, with all previous states cleared. This allows you to test your app's behavior from a clean slate.
- Focusing on App Mounting: Typically, you'll only perform this reset when your app initially loads, not every time the URL changes. This approach ensures that you're testing the app's behavior when it first starts up.
- Reset Partially: Not every state need to be cleared when we are using reset flag feature. We can reset state selectively, to make testing easier and faster. for example we might want to keep the login state of user while testing a feature which works for logged-in users only.
- Reset Implementation: Normally its not available out of the box, but there are plugins to help you out to reset your states.
By following this method, you can effectively debug stateful front-end apps. It simplifies the testing process and provides you with a reliable way to identify and fix issues without impacting your users.
React Example :
In React, you can implement reset flags using the react-router
library. Below is a simplified example:
import React, { useEffect } from 'react';
import { useLocation } from 'react-router-dom';
function App() {
const location = useLocation();
useEffect(() => {
// Check if the reset flag exists in the URL
const resetFlag = new URLSearchParams(location.search).get('reset');
if (resetFlag) {
// Perform a reset of your app's state here
}
}, []);
// Rest of your app components and logic
return (
// Your JSX here
);
}
export default App;
In this React example, we use useLocation
from react-router-dom
to access the current URL. We then check if a reset
parameter exists in the URL query string. If it does, you can perform a full reset of your app's state.
<template>
<!-- Your app template here -->
</template>
<script>
export default {
created() {
// Check if the reset flag exists in the URL
const resetFlag = this.$route.query.reset;
if (resetFlag) {
// Perform a full reset of your app's state here
}
},
// Rest of your Vue.js component logic
};
</script>
In this Vue.js example, we access the current route's query parameters using this.$route.query
and check if a reset
parameter exists. If it does, you can reset your app's state accordingly.
In conclusion, debugging stateful frontend apps doesn't have to be a headache. With the help of reset flags in your app's URL, you can streamline your testing process, making your development work smoother and ensuring a seamless user experience. Debug like a pro, catch those bugs early, and deliver a top-notch frontend app to your audience.
Bonus: How do I reset the State?
To make use of this concept, You have to implement a reset mechanism in your state store. Below is a sample implementation in Vuex
import Vue from "vue";
//initial state
function initialState() {
return {
list: {},
fetched_at: 0,
};
}
const state = initialState();
// getters
const getters = {
list: (state) => state.list,
};
//actions
const actions = {
async list(context, payload) {},
async fetch(context, payload) {},
async create(context, payload) {},
};
//mutations
const mutations = {
reset(state) {
// acquire initial state
const s = initialState();
Object.keys(s).forEach((key) => {
state[key] = s[key];
});
},
};
export default {
namespaced: true,
state,
getters,
actions,
mutations,
};
Have you tried using reset flags in your frontend development work? I love to hear your thoughts and experiences! Please share your comments and tips below.